623. 在二叉树中增加一行
给定一个二叉树的根 root
和两个整数 val
和 depth
,在给定的深度 depth
处添加一个值为 val
的节点行。注意,根节点 root
位于深度 1
。
加法规则如下:
给定整数 depth
,对于深度为 depth - 1
的每个非空树节点 cur
,
创建两个值为 val
的树节点作为 cur
的左子树根和右子树根。
cur
原来的左子树应该是新的左子树根的左子树。
cur
原来的右子树应该是新的右子树根的右子树。
如果 depth == 1
意味着 depth - 1
根本没有深度,那么创建一个树节点,值 val
作为整个原始树的新根,而原始树就是新根的左子树。
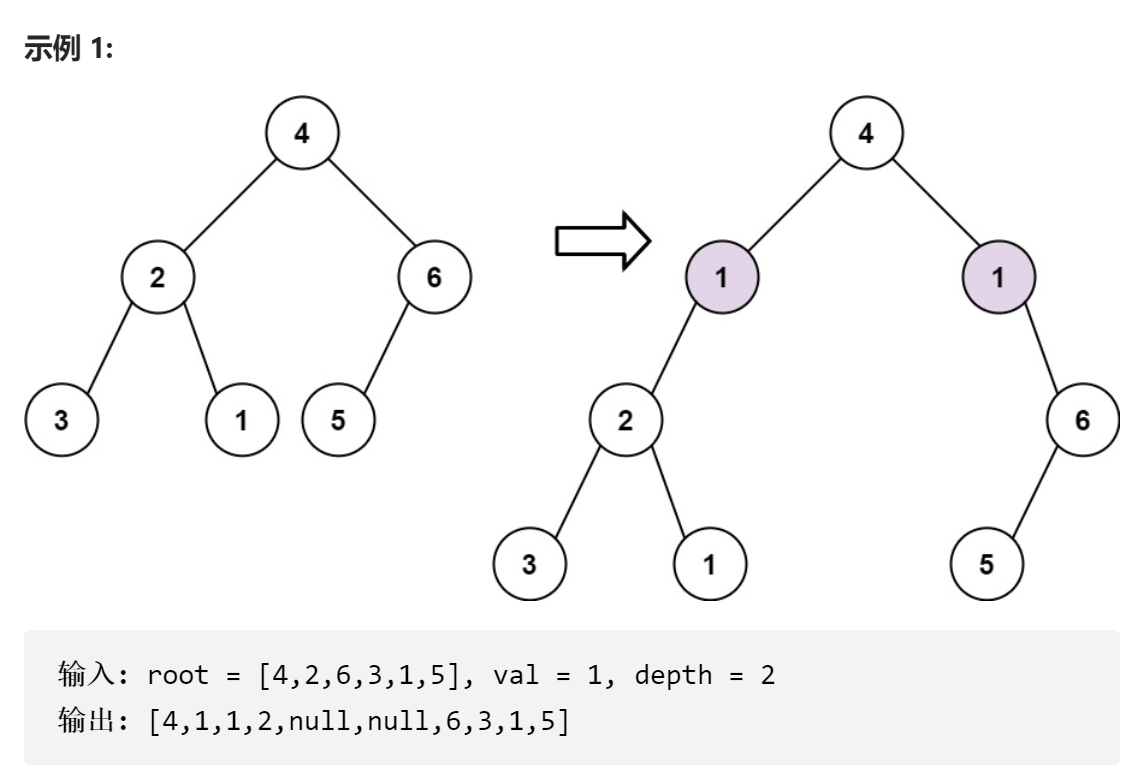
层序遍历
层序遍历记录下深度,简单
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
| func addOneRow(root *TreeNode, val int, depth int) *TreeNode {
if depth == 1 {
return &TreeNode{Val: val, Left: root}
}
queue := []*TreeNode{root}
curDepth := 1
curLevelCnt := 1
nextLevelCnt := 0
for curDepth < depth-1 {
// 出队
curNode := queue[0]
queue = queue[1:]
if curNode.Left != nil {
queue = append(queue, curNode.Left)
nextLevelCnt++
}
if curNode.Right != nil {
queue = append(queue, curNode.Right)
nextLevelCnt++
}
curLevelCnt--
if curLevelCnt == 0 {
// 下一层
curDepth++
curLevelCnt = nextLevelCnt
nextLevelCnt = 0
}
}
for _, node := range queue {
node.Left = &TreeNode{Val: val, Left: node.Left}
node.Right = &TreeNode{Val: val, Right: node.Right}
}
return root
}
|
DFS

1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
| func addOneRow(root *TreeNode, val int, depth int) *TreeNode {
if root == nil {
return nil
}
if depth == 1 {
return &TreeNode{Val: val, Left: root}
}
if depth == 2 {
root.Left = &TreeNode{Val: val, Left: root.Left}
root.Right = &TreeNode{Val: val, Right: root.Right}
} else {
root.Left = addOneRow(root.Left, val, depth-1)
root.Right = addOneRow(root.Right, val, depth-1)
}
return root
}
|