剑指 Offer 68 - I. 二叉搜索树的最近公共祖先
给定一个二叉搜索树, 找到该树中两个指定节点的最近公共祖先。(一个节点也可以是它自己的祖先)
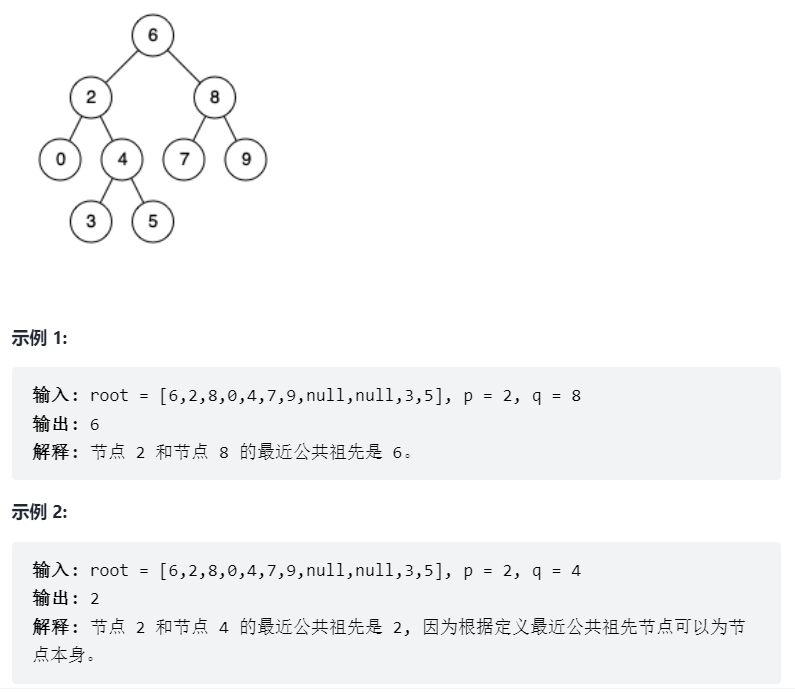
两次遍历


1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| func getPath(root, target *TreeNode) (path []*TreeNode) {
// 获取从根节点到目标节点的路径结点数组
node := root
for node != target {
path = append(path, node)
if target.Val < node.Val {
node = node.Left
} else {
node = node.Right
}
}
path = append(path, node)
return
}
func lowestCommonAncestor(root, p, q *TreeNode) (ancestor *TreeNode) {
pathP := getPath(root, p)
pathQ := getPath(root, q)
for i := 0; i < len(pathP) && i < len(pathQ) && pathP[i] == pathQ[i]; i++ {
ancestor = pathP[i]
}
return
}
|
一次遍历

1
2
3
4
5
6
7
8
9
10
11
12
| func lowestCommonAncestor(root, p, q *TreeNode) (ancestor *TreeNode) {
ancestor = root
for {
if p.Val < ancestor.Val && q.Val < ancestor.Val {
ancestor = ancestor.Left
} else if p.Val > ancestor.Val && q.Val > ancestor.Val {
ancestor = ancestor.Right
} else {
return
}
}
}
|